Koa js
What is Koa js?

In previous articles we discussed Node-js. But today I am going to give an introduction about koa js and what are the steps that we need to follow in order to create an application using koa js.
Koa-js is an open source Node-js web framework that was developed by the creators of express-js. On koa js official website they mention that the
koa framework aims to be a smaller, more expressive, and more robust foundation for web applications and APIs.
Koa-js uses asynchronous functions to eliminate callbacks and to improve error management.
Koa-js contains a set of methods for writing servers quickly; because of that koa does not contain any middleware within its core.
Koa js features
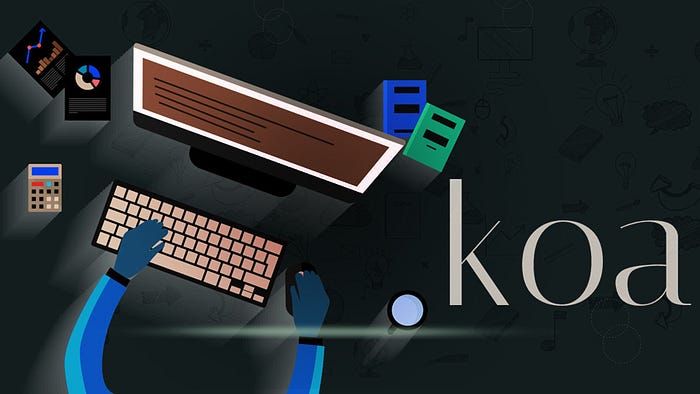
Like all the other JavaScript frameworks, koa js also has its own unique characteristics that make koa js more reliable and efficient.
Koa js is modern
Koa-js build is based on ES6, that means using koa js we can develop complex applications easily because within the koa js it contains many modern classes and modules that help developers to create and maintain their application in better way.
Koa has a small footprint
Koa-js is a very small framework (600 lines of code) and a very thin layer of abstraction to create server side applications. koa js also contains many npm packages that provide different services to the framework.
Huge community support.
As I mentioned earlier, koa js is an open source framework. Because of that, there are many developers that are improving and solving issues related to koa .So if we come up with a new problem, we call always get support from the koa community.
Better error handling
As I mentioned in the first section, koa-js uses asynchronous functions to manage errors in a better way. Also, koa js contains a build-in catchall for catching errors. So, because of that, the percentage that a website can crash has been reduced.
It uses ES6 generators
Koa.js uses ES6 generators to simplify synchronous programming and facilitate the flow of controls. And also ES6 generators can also be used as functions to control code execution on the same stack.
Who use koa js?
Many companies use Koa.js framework for their websites and web APIs.
These are some of them
1. Paralect
2. Pubu
3. Bulb
4. GAPO
5. Clovis
Koa vs express
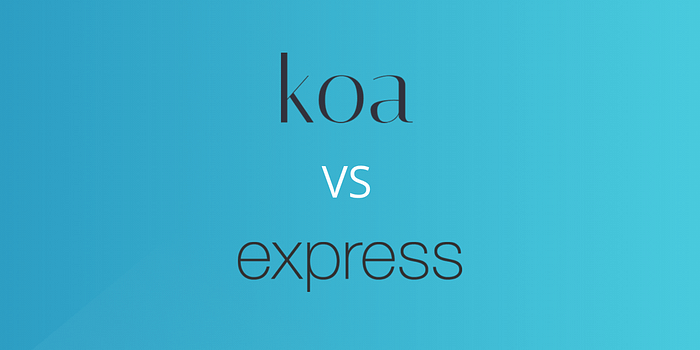
The main difference between Koa-js and Express-js is how they handle middleware. Express contains routing and templates in the application framework. On the other hand, koa requires modules for these features, therefore making it more modular or customizable.so because of that, koa js is less weighted that express js.
One of the major differences between koa and express is koa-js uses its own ctx, request ad ctx. response objects instead of the req, res in node js.
How is Koa different from Express?
o Better error handling
o Routing is not available
o No callback hell
o Koa depends less on middleware
o less weight that express
o high performance and better user Experience
Let’s Build a Simple koa server
First we need to initialize the directory using command.
npm init
Then we need to install the koa npm package using following command
npm i koa
Then in the file we can import the koa that we installed in to a variable.
const koa = require(“koa”)
Then we can create new object from that variable koa.
const app = new koa()
The app. listen()
function is used to bind and listen the connections on the specified host and port.
app.listen(3000,()=>{console.log(“server run on port 3000”)})
Then we can run the app using node index.js
command and the server run on port 3000
message will display in the console.
Let’s Create a route using koa js
First we need to install the npm package koa-router.
npm install koa-router
Then we need to import the koa-router to a variable.
const koaRouter = require('koa-router')
Then we can create a new koaRouter
object using following code
const router = new koaRouter()
Then we can specify the route url and what should be print when there is a GET
request that related to give URL
From this code we said to the server that Run this on a GET request, for the given URL.
router.get(‘home’, ‘/’, (context) => {
context.body = “Welcome to AF Blogs”
})
Then we need to register the routes to the application using the given code segment.
app.use(router.routes()).use(router.allowedMethods())
Now if we send a request that matches to the http type and URL specified in this route it will render the information that contains in the response object.
Then we can start the sever again.
node <file name>
Then if we send a request to the specified URL
in the koa js router it will display the results accordingly.
I hope you found this useful. I would like to hear from you so feel free to drop a comment or connect with me via LinkedIn.